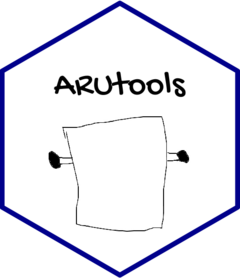
Create a look around expression and add it to an existing regular expression
Source:R/create_patterns.R
create_lookaround.Rd
Lookarounds allow you to position a regular expression to more specificity.
Examples
# Here is a string with three patterns of digits
text <- "cars123ruin456cities789"
# To extract the first one we can use this pattern
stringr::str_extract(text, "\\d{3}")
#> [1] "123"
# or
create_lookaround("\\d{3}", "cars", "before") |>
stringr::str_extract(string=text)
#> [1] "123"
# To exclude the first one we can write
create_lookaround("\\d{3}", "cars", "before", negate=TRUE) |>
stringr::str_extract_all(string=text)
#> [[1]]
#> [1] "456" "789"
#>
# To extract the second one we can write
create_lookaround("\\d{3}", "ruin", "before") |>
stringr::str_extract(string=text)
#> [1] "456"
# or
create_lookaround("\\d{3}", "cities", "after") |>
stringr::str_extract(string=text)
#> [1] "456"